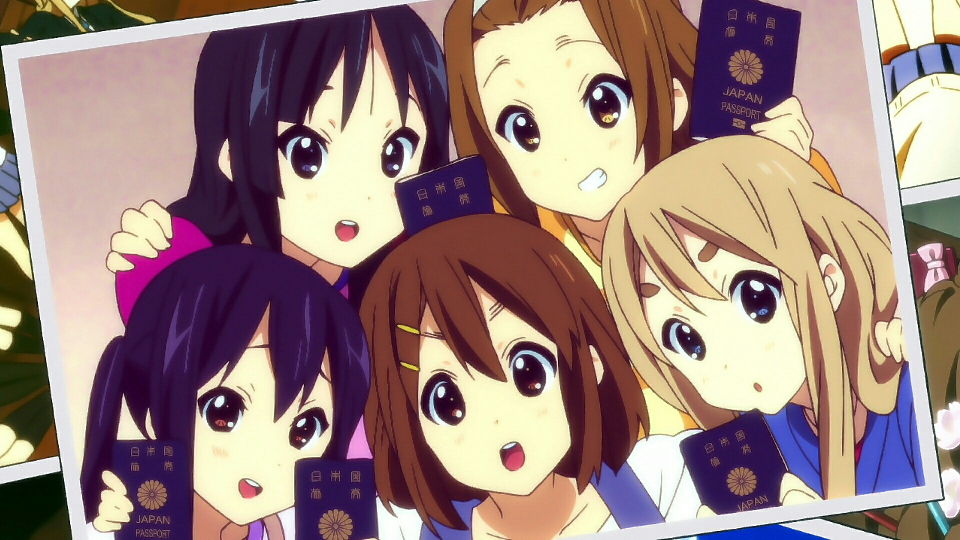
04-Python基本函数及其用法
print是输出函数.作用就是输出一段内容.
1. 源码
1 |
|
2. 语法
print(<参数1>,<参数2>)
1
2
3
4print('zzy')
print(114514)
# output: zzy
# output: 114514print(<输出内容>, sep=' ', end = '\n', file = None)
1
2
3print(123,456, sep = '', end = '')
print(789)
# output: 123456789
3. 源码解析
@overload
@overload
是Python的装饰器,它的作用是声明函数的重载.它可以用来为函数定义不同的签名(即参数列表),从而在不同的情况下调用不同的代码.详见后续装饰器的章节values
*values: object
:*values
: 参数名,可变长度参数,通常以*
开头,把收集到的参数打包为一个元组(Tuple),这里是打包为一个名为value
的元组.object
: 类型注解,指定了参数的类型.object
表示接受的参数类型是任意的
sep
sep: str | None = " "
:sep
: 表示输出时的分隔符.str | None
: 类型注解,表示可以是字符串类型或者None
类型." "
: 默认值.一个空格字符串" "
.
end
end: str | None = "\n"
:end
: 参数名,表示输出结束时要附加的字符串.str | None
: 类型注解,表示可以是字符串类型或者None
类型."\n"
: 默认值.换行符"\n"
.
file
file: SupportsWrite[str] | None = None
:file
:参数名,表示输出的目标文件对象.SupportsWrite[str] | None
: 类型注解,表示可以是支持写入操作的文件对象或者None
类型.None
: 默认值,表示输出到标准输出流.
flush
flush: Literal[False] = False
:flush
: 参数名,表示是否立即刷新输出缓冲区.Literal[False]
: 类型注解,表示只能取值为False
, 不能为其他值.False
: 默认值.
print() -> None: ...
- 返回值类型注解为
None
,表示该函数不返回任何值.
- 返回值类型注解为
对参数的类型注解和默认值,稍加拆解可能会更加清晰些
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
def fun(param1:str,param2:int|float):
pass
# 表示参数1要求接收的数据类型为str,参数2要求接收的数据类型为int或是float,其他类型可能会报错
def fun(param1=1):
pass
# 表示参数的默认值为 1
# 因此以sep为例
def print(sep: str | None = " "):
pass
# 可以分段拆分理解
# sep: str | None
# sep = " "
# 合并,即,类型注解为str | None,默认值为" ",
# 而不是当sep取值为None的时候,sep取默认值" "
# 因此
# sep: str | None = " " 与 sep: None | str = " " 这两种写法完全等价
案例
1 |
|
range
range() 可以表示一个数值的范围.
结合in.可以做一个简单的数值范围判断
源码
1 |
|
源码解析:
看不懂,略()
语法
range(start, stop[, step])
参数说明
- **start (可选)**:序列的起始数字。默认值为0。
- stop:序列的结束数字,但不包括在内。
range()
会生成从start
到stop-1
的整数序列。 - **step (可选)**:两个数之间的差(步长)。默认值为1。
返回值
range()
函数返回一个 range
对象,该对象是一个迭代器,可以生成一系列整数。
input
源码
1 |
|
参数
__prompt
: 输入的内容吗
返回值
字符串